Dart is a programming language developed by Google. It is designed for building web, mobile, and server applications. Dart is known for its focus on simplicity, productivity, and performance. Dart can connect to various types of databases through different libraries and packages. The choice of database depends on the specific requirements of your project.
The problem is, mostly guidelines that available in Youtube or websites explain about connecting database inside frameworks like Flutter or Aqueduct. It’s common to use the database solutions that are well-supported and integrated with those frameworks but you need also a high hardware resource. I still can’t find a website explain about how to connect Dart outside the framework.
So, I will explain in this article about it. I assume that you’ve already familiar with MySQL dan Dart. I use Dart SDK version: 3.1.5 (stable) (Tue Oct 24 04:57:17 2023 +0000) on “windows_x64” and mysql Ver 15.1 Distrib 10.4.28-MariaDB, for Win64 (AMD64), source revision c8f2e9a5c0ac5905f28b050b7df5a9ffd914b7e7.
I’m sure you are questioning why I wrote the code in Windows instead of Linux. Actually, dart and Xampp can run both in Windows and Linux. And the moment, I’m in the middle of working with Visual Code in Windows, so why not.
Step 1. Create a new user, make a database and table in MySQL.
When I use ‘root’ user that has no password, it didn’t work. So I create a new user because I don’t want to change the default configuration in MySQL. You can create your own database.


Step 2.Download the MySQL library in Dart.
Open file pubspec.yaml, add
dependencies: mysql1: ^0.19.0
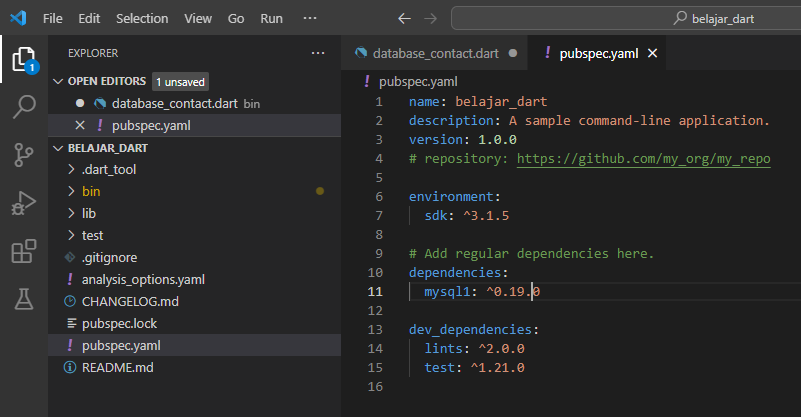
On the terminal, type:
c:\> dart pub get Resolving dependencies... (1.1s) js 0.6.7 (0.7.0 available) lints 2.1.1 (3.0.0 available) mysql1 0.19.2 (0.20.0 available) web_socket_channel 2.4.0 (2.4.1 available) Got dependencies! c:\>
Step 3. Write the dart code.
import 'package:mysql1/mysql1.dart'; Future createConnection() async { final MySqlConnection connection = await MySqlConnection.connect(ConnectionSettings( host: 'localhost', port: 3306, user: 'testing', db: 'contact', password:'testing' )); return connection; } Future fetchData() async { final MySqlConnection connection = await createConnection(); Results results = await connection.query('SELECT * FROM person'); for (var row in results) { print('${row['id']}' '${row['name']}' '${row['mobile']}' '${row['category']}' '${row['city']}'); } } void main() { fetchData(); }Continue Reading »